Kotlin Engine
This engine generates models in Kotlin code. It relies on the following kotlinx libraries:
- kotlinx.serialization: for JSON serialization
- kotlinx.datetime: for date/datetime data types
Getting Started
Once you add a Kotlin engine, you should navigate to the engine config
and set a package for the Kotlin models, just like we did in the screenshot below.
Of course your package probably won't be io.onegen.models
.
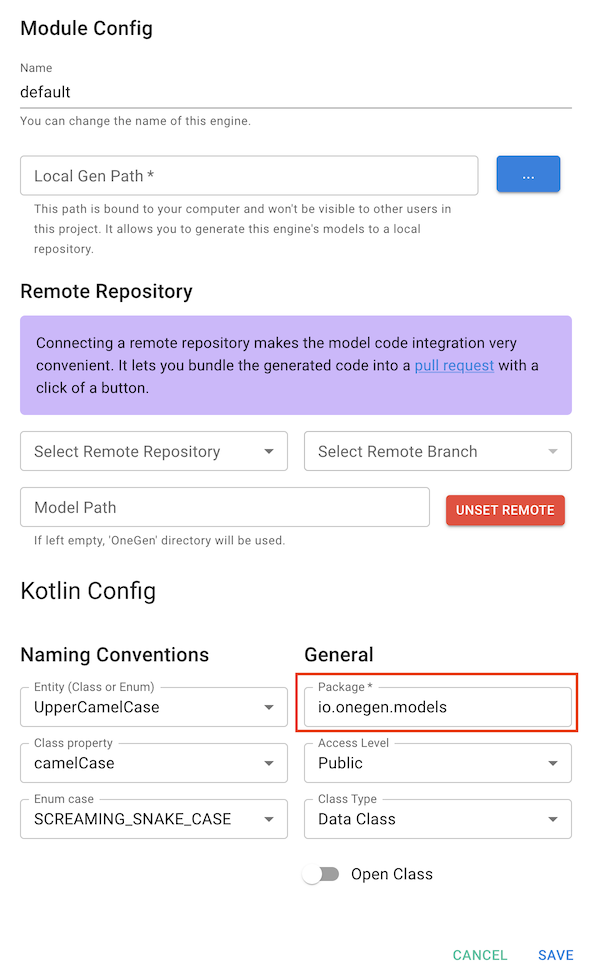
Gradle Config
Let's take a look at what needs to be configured to get the Kotlin models working in your project.
Add dependencies
As mentioned above, Kotlin engine relies on a few dependencies. Add them to your gradle's dependencies block.
dependencies {
// other dependencies in your project
implementation 'org.jetbrains.kotlinx:kotlinx-datetime:0.4.0'
implementation 'org.jetbrains.kotlinx:kotlinx-serialization-json:1.4.0'
}
Add the serialization plugin
Add the following line to your plugins block.
plugins {
// other plugins in your project
id 'org.jetbrains.kotlin.plugin.serialization' version '1.6.10'
}
Then apply the plugin by adding:
apply plugin: 'kotlinx-serialization'
Important: Make sure to update the versions kotlinx-datetime and kotlinx-serialization versions.
Hello Kotlin Example
We published an example repository where we generated Kotlin models of a simple Notes app. You can see the necessary gradle setup as well as some model code.
Data Types
Standard
Since java doesn't support unsigned data types, all unsigned data types are expressed as signed data types.
Design | Kotlin |
---|---|
bool | Boolean |
int | Int |
int8 | Byte |
int16 | Short |
int32 | Int |
int64 | Long |
uint | UInt |
uint8 | UByte |
uint16 | UShort |
uint32 | UInt |
uint64 | ULong |
float | Float |
double | Double |
string | String |
char | Char |
byte | Byte |
bytea | ByteArray |
date | LocalDate |
datetime | LocalDateTime |
Complex
Name | Kotlin Type |
---|---|
Array | List |
Map | Map |
Why is Android Room not supported?
We'd love to support the Room database, but its relationship implementation is quite restrictive. We ultimately decided it was out of our scope, we found a way to support you though.
If your project uses the Room database, there are two ways to integrate OneGen models:
a) Using the @Embedded feature
Create wrapper models and use the @Embedded
feature to embed the OneGen models.
b) Using Inheritance
Go to the Kotlin config and in Kotlin's General section, do the following:
- In Class Type select box, pick Regular
- Check the Open Class switch
- Now implement your Room models by inheriting from OneGen models